Summary: Don’t use the GET method in Ajax Apps, if you can void it, because IE7 craps out with more than 2032 characters in a get string.
Here’s the page that craps out at 2033 characters with IE7:
http://www.codebelay.com/status122/?i_limit=2033
You won’t see the error with other browsers.
Here’s the page that’s okay:
http://www.codebelay.com/status122/?i_limit=2000
What’s going on here?
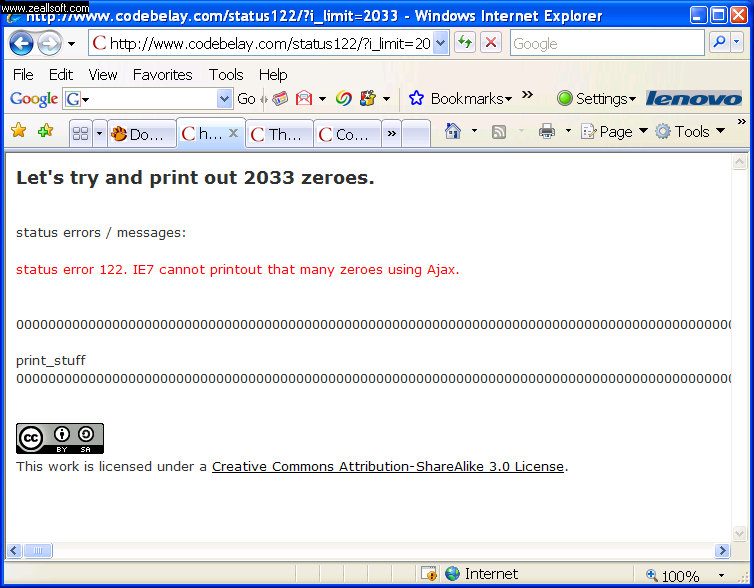
Sometimes you’ll write a piece of Javascript that uses prototype and looks like this:
var url = '/status122/listener/?stuff=' + encodeURIComponent($('js_stuff').innerHTML);
// This is where we send our raw ratings for parsing
var ajax = new Ajax.Updater(
'jskitdecoded',
url,
{
method: 'get',
onComplete: showResponse
}
);
If you print http.status you get an HTTP Status of 122. WTF?
What’s going on here is that IE7 sets a limit of 2032 characters on GET strings, so you have to do a POST instead like so:
var getvar = encodeURIComponent($('js_stuff').innerHTML);
var url = '/status122/listener/';
// This is where we send our raw data for parsing
// If we use method: 'get', IE7 will return a 122, but
// b/c Firefox is RFC2616 compliant and realizes that
// there is no minimum length for a URI, we get success.
// Here we use method: 'post' b/c IE7 is lame.
var ajax = new Ajax.Updater(
'jskitdecoded',
url,
{
method: 'post',
postBody: 'stuff=' + getvar,
onComplete: showResponse
}
);
I hope this helps.
What Ajax quirks have you run into?